Note
Go to the end to download the full example code.
Empirical Orthogonal Function (EOF) and Maximum Covariance Analysis (MCA)#
Before proceeding with all the steps, first import some necessary libraries and packages
import xarray as xr
import easyclimate as ecl
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
Empirical Orthogonal Function#
Read raw precipitation and temperature data
t_data = xr.open_dataset("t_ERA5_1982-2022_N80.nc").t.sel(time = slice("1982-01-01", "2020-12-31")).sortby("lat")
precip_data = xr.open_dataset("precip_ERA5_1982-2020_N80.nc").precip.sel(time = slice("1982-01-01", "2020-12-31")).sortby("lat")
Tip
You can download following datasets here:
Be limited to the East Asia region and conduct empirical orthogonal function (EOF) analysis on it
precip_data_EA = precip_data.sel(lon = slice(105, 130), lat = slice(20, 40))
model = ecl.eof.get_EOF_model(precip_data_EA, lat_dim = 'lat', lon_dim = 'lon', remove_seasonal_cycle_mean = True, use_coslat = True)
eof_analysis_result = ecl.eof.calc_EOF_analysis(model)
eof_analysis_result.to_netcdf("eof_analysis_result.nc")
Load the analyzed data
eof_analysis_result = xr.open_dataset("eof_analysis_result.nc")
eof_analysis_result
<xarray.Dataset> Size: 77kB Dimensions: (lat: 18, lon: 22, mode: 10, time: 468) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 80B 1 2 3 4 5 6 7 8 9 10 * time (time) datetime64[ns] 4kB 1982-01-31T18:00:00 .... month (time) int64 4kB ... Data variables: EOF (mode, lat, lon) float64 32kB ... PC (mode, time) float64 37kB ... explained_variance (mode) float64 80B ... explained_variance_ratio (mode) float64 80B ... singular_values (mode) float64 80B ...
- lat: 18
- lon: 22
- mode: 10
- time: 468
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2 3 4 5 6 7 8 9 10
array([ 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
- time(time)datetime64[ns]1982-01-31T18:00:00 ... 2020-12-...
- standard_name :
- time
- axis :
- T
array(['1982-01-31T18:00:00.000000000', '1982-02-28T18:00:00.000000000', '1982-03-31T18:00:00.000000000', ..., '2020-10-31T18:00:00.000000000', '2020-11-30T18:00:00.000000000', '2020-12-31T18:00:00.000000000'], shape=(468,), dtype='datetime64[ns]')
- month(time)int64...
- standard_name :
- time
- axis :
- T
[468 values with dtype=int64]
- EOF(mode, lat, lon)float64...
- model :
- EOF analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:14:30
- n_modes :
- 10
- center :
- False
- standardize :
- False
- use_coslat :
- True
- check_nans :
- True
- sample_name :
- sample
- feature_name :
- feature
- random_state :
- None
- compute :
- True
- solver :
- auto
- solver_kwargs :
- {}
[3960 values with dtype=float64]
- PC(mode, time)float64...
- model :
- EOF analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:14:30
- n_modes :
- 10
- center :
- False
- standardize :
- False
- use_coslat :
- True
- check_nans :
- True
- sample_name :
- sample
- feature_name :
- feature
- random_state :
- None
- compute :
- True
- solver :
- auto
- solver_kwargs :
- {}
[4680 values with dtype=float64]
- explained_variance(mode)float64...
- model :
- EOF analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:14:30
- n_modes :
- 10
- center :
- False
- standardize :
- False
- use_coslat :
- True
- check_nans :
- True
- sample_name :
- sample
- feature_name :
- feature
- random_state :
- None
- compute :
- True
- solver :
- auto
- solver_kwargs :
- {}
[10 values with dtype=float64]
- explained_variance_ratio(mode)float64...
- model :
- EOF analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:14:30
- n_modes :
- 10
- center :
- False
- standardize :
- False
- use_coslat :
- True
- check_nans :
- True
- sample_name :
- sample
- feature_name :
- feature
- random_state :
- None
- compute :
- True
- solver :
- auto
- solver_kwargs :
- {}
[10 values with dtype=float64]
- singular_values(mode)float64...
- model :
- EOF analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:14:30
- n_modes :
- 10
- center :
- False
- standardize :
- False
- use_coslat :
- True
- check_nans :
- True
- sample_name :
- sample
- feature_name :
- feature
- random_state :
- None
- compute :
- True
- solver :
- auto
- solver_kwargs :
- {}
[10 values with dtype=float64]
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2, 3, 4, 5, 6, 7, 8, 9, 10], dtype='int64', name='mode'))
- timePandasIndex
PandasIndex(DatetimeIndex(['1982-01-31 18:00:00', '1982-02-28 18:00:00', '1982-03-31 18:00:00', '1982-04-30 18:00:00', '1982-05-31 18:00:00', '1982-06-30 18:00:00', '1982-07-31 18:00:00', '1982-08-31 18:00:00', '1982-09-30 18:00:00', '1982-10-31 18:00:00', ... '2020-03-31 18:00:00', '2020-04-30 18:00:00', '2020-05-31 18:00:00', '2020-06-30 18:00:00', '2020-07-31 18:00:00', '2020-08-31 18:00:00', '2020-09-30 18:00:00', '2020-10-31 18:00:00', '2020-11-30 18:00:00', '2020-12-31 18:00:00'], dtype='datetime64[ns]', name='time', length=468, freq=None))
Draw the leading first and second modes
fig = plt.figure(figsize = (12, 8))
fig.subplots_adjust(hspace = 0.15, wspace = 0.2)
gs = fig.add_gridspec(3, 2)
proj = ccrs.PlateCarree(central_longitude = 200)
proj_trans = ccrs.PlateCarree()
axi = fig.add_subplot(gs[0:2, 0], projection = proj)
draw_data = eof_analysis_result["EOF"].sel(mode = 1)
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi = fig.add_subplot(gs[2, 0])
ecl.plot.line_plot_with_threshold(eof_analysis_result["PC"].sel(mode = 1), line_plot=False)
axi.set_ylim(-0.2, 0.2)
axi = fig.add_subplot(gs[0:2, 1], projection = proj)
draw_data = eof_analysis_result["EOF"].sel(mode = 2)
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi = fig.add_subplot(gs[2, 1])
ecl.plot.line_plot_with_threshold(eof_analysis_result["PC"].sel(mode = 2), line_plot=False)
axi.set_ylim(-0.2, 0.2)
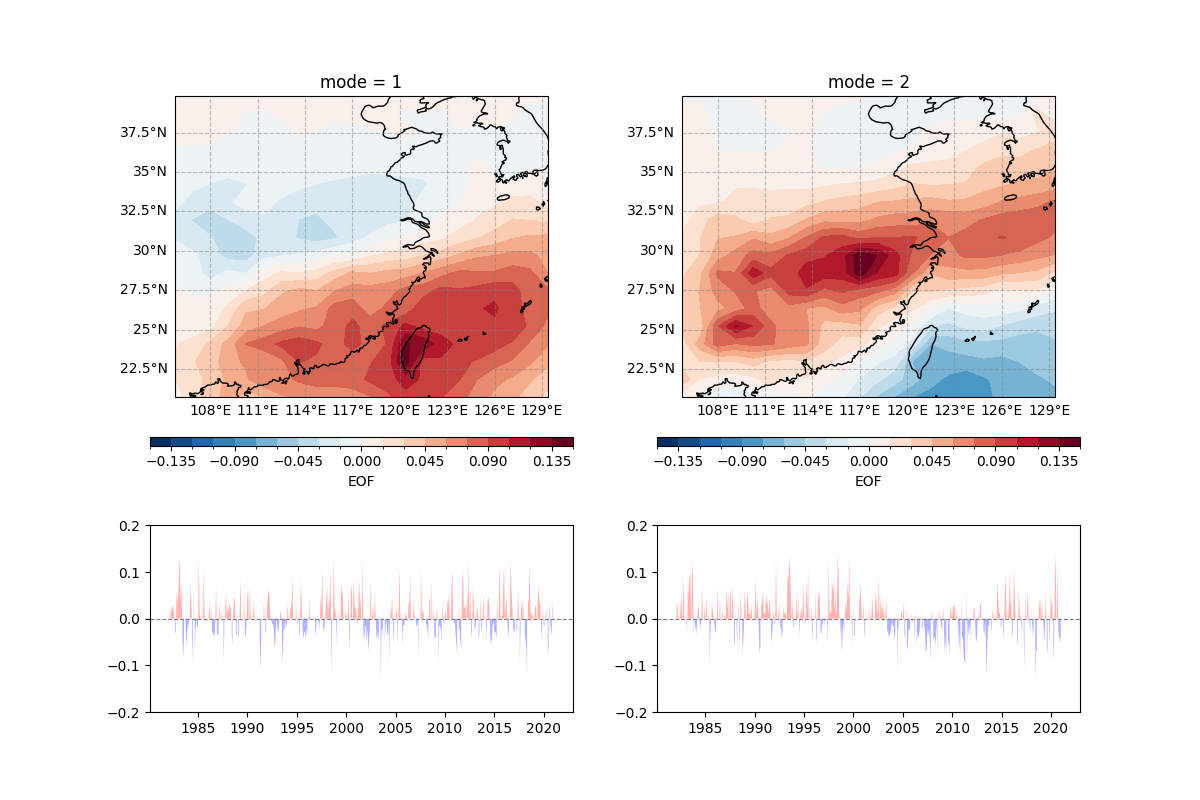
(-0.2, 0.2)
Rotated Empirical Orthogonal Function#
Here, we conduct rotated empirical orthogonal function (REOF) analysis on it
model = ecl.eof.get_REOF_model(precip_data_EA, lat_dim = 'lat', lon_dim = 'lon', remove_seasonal_cycle_mean = True, use_coslat = True)
reof_analysis_result = ecl.eof.calc_REOF_analysis(model)
reof_analysis_result.to_netcdf("reof_analysis_result.nc")
Now load the data
reof_analysis_result = xr.open_dataset("reof_analysis_result.nc")
reof_analysis_result
<xarray.Dataset> Size: 22kB Dimensions: (lat: 18, lon: 22, mode: 2, time: 468) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 16B 1 2 * time (time) datetime64[ns] 4kB 1982-01-31T18:00:00 .... month (time) int64 4kB ... Data variables: EOF (mode, lat, lon) float64 6kB ... PC (mode, time) float64 7kB ... explained_variance (mode) float64 16B ... explained_variance_ratio (mode) float64 16B ... singular_values (mode) float64 16B ...
- lat: 18
- lon: 22
- mode: 2
- time: 468
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- time(time)datetime64[ns]1982-01-31T18:00:00 ... 2020-12-...
- standard_name :
- time
- axis :
- T
array(['1982-01-31T18:00:00.000000000', '1982-02-28T18:00:00.000000000', '1982-03-31T18:00:00.000000000', ..., '2020-10-31T18:00:00.000000000', '2020-11-30T18:00:00.000000000', '2020-12-31T18:00:00.000000000'], shape=(468,), dtype='datetime64[ns]')
- month(time)int64...
- standard_name :
- time
- axis :
- T
[468 values with dtype=int64]
- EOF(mode, lat, lon)float64...
- model :
- Rotated EOF analysis
- n_modes :
- 2
- power :
- 1
- max_iter :
- 1000
- rtol :
- 1e-08
- compute :
- True
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:18:09
[792 values with dtype=float64]
- PC(mode, time)float64...
- model :
- Rotated EOF analysis
- n_modes :
- 2
- power :
- 1
- max_iter :
- 1000
- rtol :
- 1e-08
- compute :
- True
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:18:09
[936 values with dtype=float64]
- explained_variance(mode)float64...
- model :
- Rotated EOF analysis
- n_modes :
- 2
- power :
- 1
- max_iter :
- 1000
- rtol :
- 1e-08
- compute :
- True
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:18:09
[2 values with dtype=float64]
- explained_variance_ratio(mode)float64...
- model :
- Rotated EOF analysis
- n_modes :
- 2
- power :
- 1
- max_iter :
- 1000
- rtol :
- 1e-08
- compute :
- True
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:18:09
[2 values with dtype=float64]
- singular_values(mode)float64...
- model :
- Rotated EOF analysis
- n_modes :
- 2
- power :
- 1
- max_iter :
- 1000
- rtol :
- 1e-08
- compute :
- True
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-04-25 22:18:09
[2 values with dtype=float64]
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
- timePandasIndex
PandasIndex(DatetimeIndex(['1982-01-31 18:00:00', '1982-02-28 18:00:00', '1982-03-31 18:00:00', '1982-04-30 18:00:00', '1982-05-31 18:00:00', '1982-06-30 18:00:00', '1982-07-31 18:00:00', '1982-08-31 18:00:00', '1982-09-30 18:00:00', '1982-10-31 18:00:00', ... '2020-03-31 18:00:00', '2020-04-30 18:00:00', '2020-05-31 18:00:00', '2020-06-30 18:00:00', '2020-07-31 18:00:00', '2020-08-31 18:00:00', '2020-09-30 18:00:00', '2020-10-31 18:00:00', '2020-11-30 18:00:00', '2020-12-31 18:00:00'], dtype='datetime64[ns]', name='time', length=468, freq=None))
fig = plt.figure(figsize = (12, 8))
fig.subplots_adjust(hspace = 0.15, wspace = 0.2)
gs = fig.add_gridspec(3, 2)
proj = ccrs.PlateCarree(central_longitude = 200)
proj_trans = ccrs.PlateCarree()
axi = fig.add_subplot(gs[0:2, 0], projection = proj)
draw_data = reof_analysis_result["EOF"].sel(mode = 1)
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi = fig.add_subplot(gs[2, 0])
ecl.plot.line_plot_with_threshold(reof_analysis_result["PC"].sel(mode = 1), line_plot=False)
axi.set_ylim(-0.2, 0.2)
axi = fig.add_subplot(gs[0:2, 1], projection = proj)
draw_data = reof_analysis_result["EOF"].sel(mode = 2)
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi = fig.add_subplot(gs[2, 1])
ecl.plot.line_plot_with_threshold(reof_analysis_result["PC"].sel(mode = 2), line_plot=False)
axi.set_ylim(-0.2, 0.2)
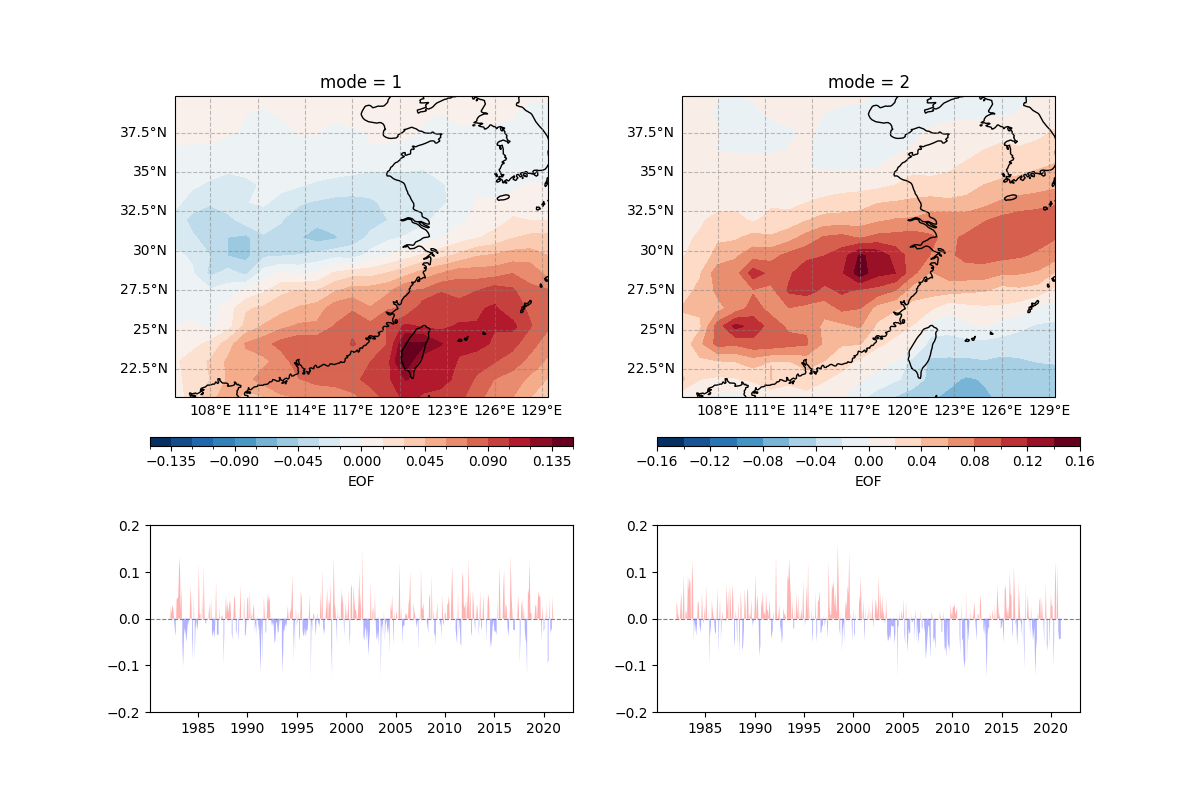
(-0.2, 0.2)
Maximum Covariance Analysis#
Be limited to the East Asia region for precipitation and temperature.
precip_data_EA = precip_data.sel(lon = slice(105, 130), lat = slice(20, 40))
t_data_EA = t_data.sel(lon = slice(105, 130), lat = slice(20, 40)).sel(level = 1000)
Maximum Covariance Analysis (MCA) between two data sets.
mca_model = ecl.eof.get_MCA_model(precip_data_EA, t_data_EA, lat_dim="lat", lon_dim="lon", n_modes=2, use_coslat=True,random_state=0)
mca_analysis_result = ecl.eof.calc_MCA_analysis(mca_model)
mca_analysis_result.to_zarr("mca_analysis_result.zarr")
Now load the data
mca_analysis_result = ecl.open_datanode("./mca_analysis_result.zarr")
mca_analysis_result
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, mode: 2, lon: 22) Coordinates: * lat (lat) float64 144B 20.75 21.87 22.99 24.11 ... 37.57 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 108.0 109.1 ... 127.1 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: left_EOF (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- mode: 2
- lon: 22
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- left_EOF(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- code :
- 228
- table :
- 128
- CDI_grid_type :
- gaussian
- CDI_grid_num_LPE :
- 80
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 21.87 22.99 24.11 ... 37.57 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 108.0 109.1 ... 127.1 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: right_EOF (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- right_EOF(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- standard_name :
- air_temperature
- long_name :
- Temperature
- units :
- K
- CDI_grid_type :
- gaussian
- CDI_grid_num_LPE :
- 80
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 11kB Dimensions: (mode: 2, time: 468) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 * time (time) datetime64[ns] 4kB 1982-01-31T18:00:00 ... 2020-12-31T18:... Data variables: left_PC (mode, time) float64 7kB dask.array<chunksize=(2, 468), meta=np.ndarray>
- mode: 2
- time: 468
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- time(time)datetime64[ns]1982-01-31T18:00:00 ... 2020-12-...
- standard_name :
- time
- axis :
- T
array(['1982-01-31T18:00:00.000000000', '1982-02-28T18:00:00.000000000', '1982-03-31T18:00:00.000000000', ..., '2020-10-31T18:00:00.000000000', '2020-11-30T18:00:00.000000000', '2020-12-31T18:00:00.000000000'], shape=(468,), dtype='datetime64[ns]')
- left_PC(mode, time)float64dask.array<chunksize=(2, 468), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 7.31 kiB 7.31 kiB Shape (2, 468) (2, 468) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
- timePandasIndex
PandasIndex(DatetimeIndex(['1982-01-31 18:00:00', '1982-02-28 18:00:00', '1982-03-31 18:00:00', '1982-04-30 18:00:00', '1982-05-31 18:00:00', '1982-06-30 18:00:00', '1982-07-31 18:00:00', '1982-08-31 18:00:00', '1982-09-30 18:00:00', '1982-10-31 18:00:00', ... '2020-03-31 18:00:00', '2020-04-30 18:00:00', '2020-05-31 18:00:00', '2020-06-30 18:00:00', '2020-07-31 18:00:00', '2020-08-31 18:00:00', '2020-09-30 18:00:00', '2020-10-31 18:00:00', '2020-11-30 18:00:00', '2020-12-31 18:00:00'], dtype='datetime64[ns]', name='time', length=468, freq=None))
<xarray.Dataset> Size: 11kB Dimensions: (mode: 2, time: 468) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 * time (time) datetime64[ns] 4kB 1982-01-01 1982-02-01 ... 2020-12-01 Data variables: right_PC (mode, time) float64 7kB dask.array<chunksize=(2, 468), meta=np.ndarray>
- mode: 2
- time: 468
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- time(time)datetime64[ns]1982-01-01 ... 2020-12-01
- standard_name :
- time
- long_name :
- time
- axis :
- T
array(['1982-01-01T00:00:00.000000000', '1982-02-01T00:00:00.000000000', '1982-03-01T00:00:00.000000000', ..., '2020-10-01T00:00:00.000000000', '2020-11-01T00:00:00.000000000', '2020-12-01T00:00:00.000000000'], shape=(468,), dtype='datetime64[ns]')
- right_PC(mode, time)float64dask.array<chunksize=(2, 468), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 7.31 kiB 7.31 kiB Shape (2, 468) (2, 468) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
- timePandasIndex
PandasIndex(DatetimeIndex(['1982-01-01', '1982-02-01', '1982-03-01', '1982-04-01', '1982-05-01', '1982-06-01', '1982-07-01', '1982-08-01', '1982-09-01', '1982-10-01', ... '2020-03-01', '2020-04-01', '2020-05-01', '2020-06-01', '2020-07-01', '2020-08-01', '2020-09-01', '2020-10-01', '2020-11-01', '2020-12-01'], dtype='datetime64[ns]', name='time', length=468, freq=None))
<xarray.Dataset> Size: 68B Dimensions: (mode_x: 2, mode_y: 2) Coordinates: level int32 4B ... * mode_x (mode_x) int64 16B 1 2 * mode_y (mode_y) int64 16B 1 2 Data variables: correlation_coefficients_X (mode_x, mode_y) float64 32B dask.array<chunksize=(2, 2), meta=np.ndarray>
- mode_x: 2
- mode_y: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode_x(mode_x)int641 2
array([1, 2])
- mode_y(mode_y)int641 2
array([1, 2])
- correlation_coefficients_X(mode_x, mode_y)float64dask.array<chunksize=(2, 2), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 32 B 32 B Shape (2, 2) (2, 2) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- mode_xPandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode_x'))
- mode_yPandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode_y'))
<xarray.Dataset> Size: 68B Dimensions: (mode_x: 2, mode_y: 2) Coordinates: level int32 4B ... * mode_x (mode_x) int64 16B 1 2 * mode_y (mode_y) int64 16B 1 2 Data variables: correlation_coefficients_Y (mode_x, mode_y) float64 32B dask.array<chunksize=(2, 2), meta=np.ndarray>
- mode_x: 2
- mode_y: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode_x(mode_x)int641 2
array([1, 2])
- mode_y(mode_y)int641 2
array([1, 2])
- correlation_coefficients_Y(mode_x, mode_y)float64dask.array<chunksize=(2, 2), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 32 B 32 B Shape (2, 2) (2, 2) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- mode_xPandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode_x'))
- mode_yPandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode_y'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: covariance_fraction (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- covariance_fraction(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: cross_correlation_coefficients (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- cross_correlation_coefficients(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: fraction_variance_X_explained_by_X (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- fraction_variance_X_explained_by_X(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: fraction_variance_Y_explained_by_X (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- fraction_variance_Y_explained_by_X(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: fraction_variance_Y_explained_by_Y (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- fraction_variance_Y_explained_by_Y(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, mode: 2, lon: 22) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: left_heterogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- mode: 2
- lon: 22
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- left_heterogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 ... 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 ... 129.4 * mode (mode) int64 16B 1 2 Data variables: pvalues_of_left_heterogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- pvalues_of_left_heterogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 ... 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 ... 129.4 * mode (mode) int64 16B 1 2 Data variables: pvalues_of_right_heterogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- pvalues_of_right_heterogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: right_heterogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- right_heterogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, mode: 2, lon: 22) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: left_homogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- mode: 2
- lon: 22
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- left_homogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 ... 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 ... 129.4 * mode (mode) int64 16B 1 2 Data variables: pvalues_of_left_homogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- pvalues_of_left_homogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 ... 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 ... 129.4 * mode (mode) int64 16B 1 2 Data variables: pvalues_of_right_homogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- pvalues_of_right_homogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 7kB Dimensions: (lat: 18, lon: 22, mode: 2) Coordinates: * lat (lat) float64 144B 20.75 21.87 ... 38.69 39.81 level int32 4B ... * lon (lon) float64 176B 105.8 106.9 ... 128.2 129.4 * mode (mode) int64 16B 1 2 Data variables: right_homogeneous_patterns (mode, lat, lon) float64 6kB dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- lat: 18
- lon: 22
- mode: 2
- lat(lat)float6420.75 21.87 22.99 ... 38.69 39.81
array([20.747558, 21.869047, 22.990536, 24.112025, 25.233514, 26.355002, 27.476491, 28.597979, 29.719467, 30.840955, 31.962443, 33.083931, 34.205418, 35.326906, 36.448393, 37.56988 , 38.691366, 39.812852])
- level()int32...
- standard_name :
- air_pressure
- long_name :
- pressure_level
- units :
- millibars
- positive :
- down
- axis :
- Z
[1 values with dtype=int32]
- lon(lon)float64105.8 106.9 108.0 ... 128.2 129.4
array([105.75 , 106.875, 108. , 109.125, 110.25 , 111.375, 112.5 , 113.625, 114.75 , 115.875, 117. , 118.125, 119.25 , 120.375, 121.5 , 122.625, 123.75 , 124.875, 126. , 127.125, 128.25 , 129.375])
- mode(mode)int641 2
array([1, 2])
- right_homogeneous_patterns(mode, lat, lon)float64dask.array<chunksize=(2, 18, 22), meta=np.ndarray>
- model :
- Maximum Covariance Analysis
- software :
- xeofs
- version :
- 3.0.4
- date :
- 2025-05-08 21:40:42
- n_modes :
- 2
- center :
- ['True', 'True']
- standardize :
- ['False', 'False']
- use_coslat :
- ['True', 'True']
- check_nans :
- ['True', 'True']
- use_pca :
- ['True', 'True']
- n_pca_modes :
- [2, 2]
- pca_init_rank_reduction :
- [0.3, 0.3]
- alpha :
- [1.0, 1.0]
- sample_name :
- sample
- feature_name :
- ['feature1', 'feature2']
- random_state :
- 0
- compute :
- True
- solver :
- auto
Array Chunk Bytes 6.19 kiB 6.19 kiB Shape (2, 18, 22) (2, 18, 22) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- latPandasIndex
PandasIndex(Index([20.747558233615965, 21.86904729073011, 22.99053624854131, 24.11202509967086, 25.23351383632434, 26.355002450250566, 27.47649093269635, 28.597979274356536, 29.71946746531868, 30.840955495001804, 31.962443352088037, 33.08393102444663, 34.20541849904876, 35.32690576187227, 36.4483927977945, 37.569879590471466, 38.69136612220164, 39.81285237377123], dtype='float64', name='lat'))
- lonPandasIndex
PandasIndex(Index([ 105.75, 106.875, 108.0, 109.125, 110.25, 111.375, 112.5, 113.625, 114.75, 115.875, 117.0, 118.125, 119.25, 120.375, 121.5, 122.625, 123.75, 124.875, 126.0, 127.125, 128.25, 129.375], dtype='float64', name='lon'))
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
<xarray.Dataset> Size: 36B Dimensions: (mode: 2) Coordinates: level int32 4B ... * mode (mode) int64 16B 1 2 Data variables: squared_covariance_fraction (mode) float64 16B dask.array<chunksize=(2,), meta=np.ndarray>
- mode: 2
- level()int32...
[1 values with dtype=int32]
- mode(mode)int641 2
array([1, 2])
- squared_covariance_fraction(mode)float64dask.array<chunksize=(2,), meta=np.ndarray>
Array Chunk Bytes 16 B 16 B Shape (2,) (2,) Dask graph 1 chunks in 2 graph layers Data type float64 numpy.ndarray
- modePandasIndex
PandasIndex(Index([1, 2], dtype='int64', name='mode'))
Draw results for leading modes
fig = plt.figure(figsize = (9, 6))
fig.subplots_adjust(hspace = 0.15, wspace = 0.1)
gs = fig.add_gridspec(3, 2)
proj = ccrs.PlateCarree(central_longitude = 200)
proj_trans = ccrs.PlateCarree()
# ---------------
axi = fig.add_subplot(gs[0:2, 0], projection = proj)
draw_data = mca_analysis_result["EOF/left_EOF"].sel(mode = 2).left_EOF
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('left EOF2')
# ---------------
axi = fig.add_subplot(gs[2, 0])
ecl.plot.line_plot_with_threshold(mca_analysis_result["PC/left_PC"].sel(mode = 1).left_PC, line_plot=False)
axi.set_ylim(-0.04, 0.04)
# ---------------
axi = fig.add_subplot(gs[0:2, 1], projection = proj)
draw_data = mca_analysis_result["EOF/right_EOF"].sel(mode = 2).right_EOF
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('right EOF2')
# ---------------
axi = fig.add_subplot(gs[2, 1])
ecl.plot.line_plot_with_threshold(mca_analysis_result["PC/right_PC"].sel(mode = 1).right_PC, line_plot=False)
axi.set_ylim(-0.04, 0.04)
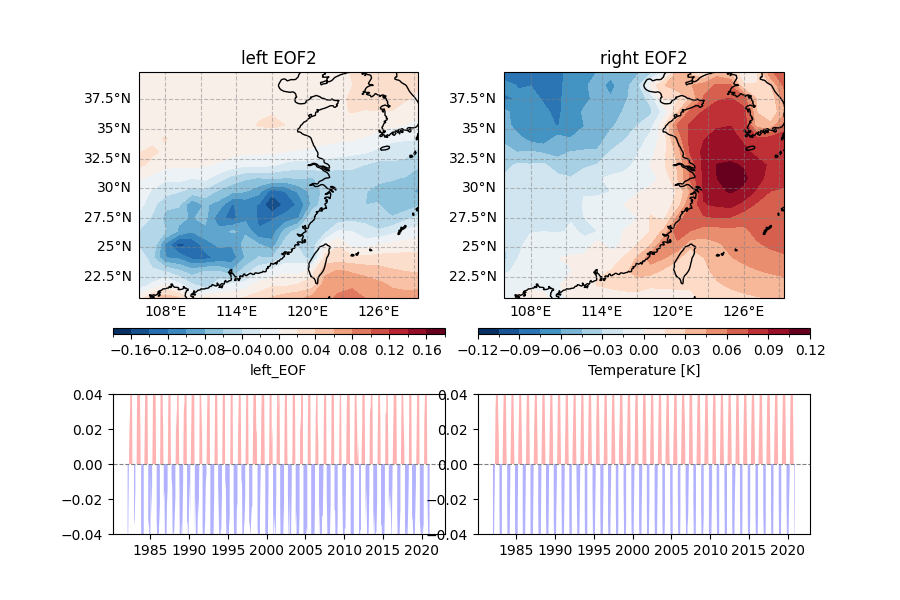
(-0.04, 0.04)
Draw the figures for both homogeneous and heterogeneous patterns
proj = ccrs.PlateCarree(central_longitude = 200)
proj_trans = ccrs.PlateCarree()
fig, ax = plt.subplots(2, 2, figsize = (9, 8), subplot_kw={"projection": proj})
# ---------------
axi = ax[0, 0]
draw_data = mca_analysis_result["heterogeneous_patterns/left_heterogeneous_patterns"].sel(mode = 2).left_heterogeneous_patterns
p_data = mca_analysis_result["heterogeneous_patterns/pvalues_of_left_heterogeneous_patterns"].sel(mode = 2).pvalues_of_left_heterogeneous_patterns
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
ecl.plot.draw_significant_area_contourf(p_data, ax = axi, thresh=0.1, transform=proj_trans)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('Left Heterogeneous Patterns')
# ---------------
axi = ax[0, 1]
draw_data = mca_analysis_result["heterogeneous_patterns/right_heterogeneous_patterns"].sel(mode = 2).right_heterogeneous_patterns
p_data = mca_analysis_result["heterogeneous_patterns/pvalues_of_right_heterogeneous_patterns"].sel(mode = 2).pvalues_of_right_heterogeneous_patterns
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
ecl.plot.draw_significant_area_contourf(p_data, ax = axi, thresh=0.1, transform=proj_trans)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('Right Heterogeneous Patterns')
# ---------------
axi = ax[1, 0]
draw_data = mca_analysis_result["homogeneous_patterns/left_homogeneous_patterns"].sel(mode = 2).left_homogeneous_patterns
p_data = mca_analysis_result["homogeneous_patterns/pvalues_of_left_homogeneous_patterns"].sel(mode = 2).pvalues_of_left_homogeneous_patterns
draw_data.plot.contourf(
ax = axi, levels = 21,
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
ecl.plot.draw_significant_area_contourf(p_data, ax = axi, thresh=0.1, transform=proj_trans)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('Left Homogeneous Patterns')
# ---------------
axi = ax[1, 1]
draw_data = mca_analysis_result["homogeneous_patterns/right_homogeneous_patterns"].sel(mode = 2).right_homogeneous_patterns
p_data = mca_analysis_result["homogeneous_patterns/pvalues_of_right_homogeneous_patterns"].sel(mode = 2).pvalues_of_right_homogeneous_patterns
draw_data.plot.contourf(
ax = axi, levels = 21,
vmax = 0.8, vmin = -0.8,
cmap = "RdBu_r",
transform = proj_trans,
cbar_kwargs={"location": "bottom", "aspect": 50, "pad" : 0.1}
)
ecl.plot.draw_significant_area_contourf(p_data, ax = axi, thresh=0.1, transform=proj_trans)
axi.coastlines()
axi.gridlines(draw_labels=["left", "bottom"], color="grey", alpha=0.5, linestyle="--")
axi.set_title('Right Homogeneous Patterns')
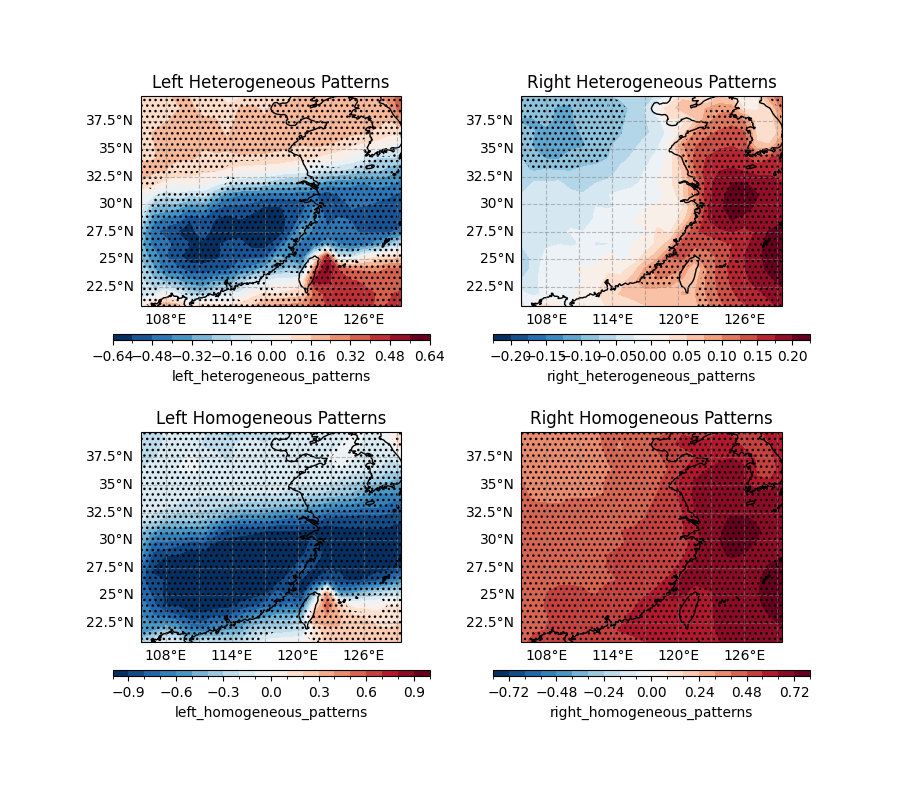
Text(0.5, 1.0, 'Right Homogeneous Patterns')
Total running time of the script: (0 minutes 6.086 seconds)