Note
Go to the end to download the full example code.
Estimate Red-noise Spectrum#
Before proceeding with all the steps, first import some necessary libraries and packages
import pooch
import xarray as xr
import numpy as np
import pandas as pd
import easyclimate as ecl
import matplotlib.pyplot as plt
from matplotlib import ticker
You can download following datasets here:
pooch.retrieve(
"https://psl.noaa.gov/data/correlation/nina34.anom.data",
known_hash=None,
fname = "nina34.anom.data",
path = ".",
)
Now we begin to read the txt file
# Read data from txt file
file_path = "nina34.anom.data"
with open(file_path, 'r') as file:
lines = file.readlines()
# Parse data
years = []
values = []
for line in lines:
# Skip empty lines or comment lines
if not line.strip() or line.startswith('#'):
continue
parts = line.split()
# Check if the first column is a year (integer)
try:
year = int(parts[0]) # Attempt to convert the first column to an integer
except ValueError:
print(f"Skipping invalid line (first column is not a year): {line.strip()}")
continue
# Check if there are 12 monthly values
if len(parts[1:]) != 12:
print(f"Skipping invalid line (missing 12 monthly values): {line.strip()}")
continue
# Convert the 12 monthly values to floats
try:
monthly_values = list(map(float, parts[1:]))
except ValueError:
print(f"Skipping invalid line (contains non-numeric data): {line.strip()}")
continue
years.append(year)
values.append(monthly_values)
# Create time index
time = pd.date_range(start=f'{years[0]}-01', periods=len(years) * 12, freq='ME')
# Flatten the data into a 1D array
flat_values = np.array(values).flatten()
# Create xarray.DataArray
nino34 = xr.DataArray(
flat_values,
dims=['time'],
coords={'time': time},
attrs={'description': 'Nino 3.4 Index', 'units': 'Celsius'}
)
# Replace -99.99 with NaN
nino34 = nino34.where(nino34 != -99.99)
Skipping invalid line (missing 12 monthly values): 1948 2025
Skipping invalid line (first column is not a year): -99.99
Skipping invalid line (first column is not a year): Nino Anom 3.4 Index using ersstv5 from CPC
Skipping invalid line (first column is not a year): https://psl.noaa.gov/data/climateindices/list/for info
Filter needed time range
nino34 = nino34.isel(time = slice(24,-12))
nino34
<xarray.DataArray (time: 900)> Size: 7kB array([-1.99, -1.69, -1.42, -1.54, -1.75, -1.27, -1.01, -0.97, -0.98, -1.03, -1.23, -1.31, -1.3 , -1.04, -0.38, -0.23, -0.01, 0. , 0.3 , 0.17, 0.51, 0.49, 0.55, 0.31, 0.13, -0.01, -0.11, -0.02, -0.14, -0.54, -0.76, -0.56, -0.36, -0.46, -0.78, -0.39, 0.2 , 0.24, 0.29, 0.22, 0.35, 0.39, 0.14, 0.09, 0.29, 0.16, 0.18, 0.41, 0.43, 0.28, -0.38, -1.17, -0.81, -0.93, -1.18, -1.43, -1.6 , -1.48, -1.13, -1.33, -0.93, -0.95, -1.06, -1.22, -1.28, -1.18, -1.15, -1.35, -1.43, -2.31, -2.45, -2.03, -1.2 , -0.99, -0.83, -0.97, -0.8 , -0.92, -1.06, -1.17, -0.98, -0.97, -1.14, -0.89, -0.51, -0.22, 0.18, 0.41, 0.62, 0.63, 0.87, 0.84, 0.72, 0.7 , 0.92, 1.3 , 1.78, 1.49, 0.98, 0.46, 0.38, 0.26, 0.03, -0.01, -0.32, -0.27, 0.05, 0.02, 0.53, 0.43, 0.19, 0.06, -0.23, -0.35, -0.86, -0.77, -0.8 , -0.48, -0.66, -0.42, -0.28, -0.46, -0.3 , -0.33, -0.25, -0.49, -0.41, -0.15, -0.28, -0.49, -0.45, -0.38, -0.31, -0.19, -0.35, -0.46, -0.18, -0.06, -0.41, -0.67, -0.94, -1.01, -0.63, -0.63, -0.59, -0.57, -0.48, -0.69, -0.89, -0.65, -0.53, -0.53, -0.78, -0.75, -0.95, -0.93, -0.78, -0.53, -0.1 , -0.03, -0.3 , -0.11, 0.49, 0.62, 0.68, 0.64, 0.77, 1.02, 0.79, 0.38, -0.26, -0.87, -1.12, -1.14, -0.96, -1.26, -1.4 , -1.35, -1.44, -1.37, ... 1.52, 1.25, 0.9 , 0.38, -0.22, -0.69, -1.07, -1.39, -1.6 , -1.69, -1.64, -1.6 , -1.54, -1.11, -0.93, -0.77, -0.52, -0.38, -0.43, -0.65, -0.8 , -1.05, -1.19, -1.06, -0.87, -0.67, -0.61, -0.5 , -0.32, 0.02, 0.25, 0.47, 0.38, 0.26, 0.16, -0.25, -0.53, -0.52, -0.25, -0.25, -0.4 , -0.42, -0.39, -0.38, -0.18, -0.2 , -0.14, -0.17, -0.49, -0.62, -0.28, 0.08, 0.32, 0.23, -0.06, -0.03, 0.29, 0.44, 0.75, 0.71, 0.51, 0.42, 0.47, 0.7 , 0.92, 1.18, 1.46, 1.93, 2.21, 2.36, 2.72, 2.66, 2.57, 2.26, 1.62, 0.91, 0.3 , -0.03, -0.48, -0.58, -0.58, -0.74, -0.76, -0.5 , -0.43, -0.08, 0.03, 0.22, 0.37, 0.34, 0.25, -0.16, -0.43, -0.56, -0.97, -0.98, -0.98, -0.78, -0.8 , -0.51, -0.2 , 0.04, 0.12, 0.09, 0.47, 0.9 , 0.9 , 0.89, 0.65, 0.71, 0.81, 0.62, 0.55, 0.45, 0.35, 0.04, 0.03, 0.48, 0.52, 0.52, 0.6 , 0.37, 0.48, 0.36, -0.27, -0.34, -0.3 , -0.59, -0.83, -1.26, -1.42, -1.15, -1. , -1. , -0.8 , -0.72, -0.46, -0.28, -0.39, -0.53, -0.55, -0.94, -0.94, -1.06, -0.94, -0.89, -0.97, -1.11, -1.11, -0.75, -0.69, -0.97, -1.07, -0.99, -0.9 , -0.85, -0.72, -0.46, -0.11, 0.14, 0.46, 0.84, 1.02, 1.35, 1.6 , 1.72, 2.02, 2.03, 1.81, 1.52, 1.13, 0.77, 0.23, 0.17, 0.04, -0.12, -0.26, -0.27, -0.25, -0.6 ]) Coordinates: * time (time) datetime64[ns] 7kB 1950-01-31 1950-02-28 ... 2024-12-31 Attributes: description: Nino 3.4 Index units: Celsius
xarray.DataArray
- time: 900
- -1.99 -1.69 -1.42 -1.54 -1.75 -1.27 ... -0.12 -0.26 -0.27 -0.25 -0.6
array([-1.99, -1.69, -1.42, -1.54, -1.75, -1.27, -1.01, -0.97, -0.98, -1.03, -1.23, -1.31, -1.3 , -1.04, -0.38, -0.23, -0.01, 0. , 0.3 , 0.17, 0.51, 0.49, 0.55, 0.31, 0.13, -0.01, -0.11, -0.02, -0.14, -0.54, -0.76, -0.56, -0.36, -0.46, -0.78, -0.39, 0.2 , 0.24, 0.29, 0.22, 0.35, 0.39, 0.14, 0.09, 0.29, 0.16, 0.18, 0.41, 0.43, 0.28, -0.38, -1.17, -0.81, -0.93, -1.18, -1.43, -1.6 , -1.48, -1.13, -1.33, -0.93, -0.95, -1.06, -1.22, -1.28, -1.18, -1.15, -1.35, -1.43, -2.31, -2.45, -2.03, -1.2 , -0.99, -0.83, -0.97, -0.8 , -0.92, -1.06, -1.17, -0.98, -0.97, -1.14, -0.89, -0.51, -0.22, 0.18, 0.41, 0.62, 0.63, 0.87, 0.84, 0.72, 0.7 , 0.92, 1.3 , 1.78, 1.49, 0.98, 0.46, 0.38, 0.26, 0.03, -0.01, -0.32, -0.27, 0.05, 0.02, 0.53, 0.43, 0.19, 0.06, -0.23, -0.35, -0.86, -0.77, -0.8 , -0.48, -0.66, -0.42, -0.28, -0.46, -0.3 , -0.33, -0.25, -0.49, -0.41, -0.15, -0.28, -0.49, -0.45, -0.38, -0.31, -0.19, -0.35, -0.46, -0.18, -0.06, -0.41, -0.67, -0.94, -1.01, -0.63, -0.63, -0.59, -0.57, -0.48, -0.69, -0.89, -0.65, -0.53, -0.53, -0.78, -0.75, -0.95, -0.93, -0.78, -0.53, -0.1 , -0.03, -0.3 , -0.11, 0.49, 0.62, 0.68, 0.64, 0.77, 1.02, 0.79, 0.38, -0.26, -0.87, -1.12, -1.14, -0.96, -1.26, -1.4 , -1.35, -1.44, -1.37, ... 1.52, 1.25, 0.9 , 0.38, -0.22, -0.69, -1.07, -1.39, -1.6 , -1.69, -1.64, -1.6 , -1.54, -1.11, -0.93, -0.77, -0.52, -0.38, -0.43, -0.65, -0.8 , -1.05, -1.19, -1.06, -0.87, -0.67, -0.61, -0.5 , -0.32, 0.02, 0.25, 0.47, 0.38, 0.26, 0.16, -0.25, -0.53, -0.52, -0.25, -0.25, -0.4 , -0.42, -0.39, -0.38, -0.18, -0.2 , -0.14, -0.17, -0.49, -0.62, -0.28, 0.08, 0.32, 0.23, -0.06, -0.03, 0.29, 0.44, 0.75, 0.71, 0.51, 0.42, 0.47, 0.7 , 0.92, 1.18, 1.46, 1.93, 2.21, 2.36, 2.72, 2.66, 2.57, 2.26, 1.62, 0.91, 0.3 , -0.03, -0.48, -0.58, -0.58, -0.74, -0.76, -0.5 , -0.43, -0.08, 0.03, 0.22, 0.37, 0.34, 0.25, -0.16, -0.43, -0.56, -0.97, -0.98, -0.98, -0.78, -0.8 , -0.51, -0.2 , 0.04, 0.12, 0.09, 0.47, 0.9 , 0.9 , 0.89, 0.65, 0.71, 0.81, 0.62, 0.55, 0.45, 0.35, 0.04, 0.03, 0.48, 0.52, 0.52, 0.6 , 0.37, 0.48, 0.36, -0.27, -0.34, -0.3 , -0.59, -0.83, -1.26, -1.42, -1.15, -1. , -1. , -0.8 , -0.72, -0.46, -0.28, -0.39, -0.53, -0.55, -0.94, -0.94, -1.06, -0.94, -0.89, -0.97, -1.11, -1.11, -0.75, -0.69, -0.97, -1.07, -0.99, -0.9 , -0.85, -0.72, -0.46, -0.11, 0.14, 0.46, 0.84, 1.02, 1.35, 1.6 , 1.72, 2.02, 2.03, 1.81, 1.52, 1.13, 0.77, 0.23, 0.17, 0.04, -0.12, -0.26, -0.27, -0.25, -0.6 ])
- time(time)datetime64[ns]1950-01-31 ... 2024-12-31
array(['1950-01-31T00:00:00.000000000', '1950-02-28T00:00:00.000000000', '1950-03-31T00:00:00.000000000', ..., '2024-10-31T00:00:00.000000000', '2024-11-30T00:00:00.000000000', '2024-12-31T00:00:00.000000000'], shape=(900,), dtype='datetime64[ns]')
- timePandasIndex
PandasIndex(DatetimeIndex(['1950-01-31', '1950-02-28', '1950-03-31', '1950-04-30', '1950-05-31', '1950-06-30', '1950-07-31', '1950-08-31', '1950-09-30', '1950-10-31', ... '2024-03-31', '2024-04-30', '2024-05-31', '2024-06-30', '2024-07-31', '2024-08-31', '2024-09-30', '2024-10-31', '2024-11-30', '2024-12-31'], dtype='datetime64[ns]', name='time', length=900, freq='ME'))
- description :
- Nino 3.4 Index
- units :
- Celsius
Calculate red noise
result_redfit = ecl.filter.calc_redfit(nino34)
result_redfit = result_redfit.assign_coords({"period_month": (result_redfit.period)/12})
result_redfit
<xarray.Dataset> Size: 22kB Dimensions: (freq: 451) Coordinates: * freq (freq) float32 2kB 0.0 0.001111 0.002222 ... 0.4978 0.4989 0.5 period (freq) float32 2kB inf 900.0 450.0 300.0 ... 2.009 2.004 2.0 period_month (freq) float32 2kB inf 75.0 37.5 25.0 ... 0.1674 0.167 0.1667 Data variables: gxx (freq) float32 2kB 1.603e-13 2.266 5.423 ... 0.01003 0.008833 gxx_corr (freq) float32 2kB 4.176 3.245 5.202 ... 0.00842 0.007062 gred_th (freq) float32 2kB 66.46 64.73 60.06 ... 0.03038 0.03038 gred (freq) float32 2kB 2.551e-12 45.19 62.61 ... 0.0362 0.038 corrFac (freq) float32 2kB 3.839e-14 0.6981 1.043 ... 1.191 1.251 chi2_80 (freq) float32 2kB 107.0 104.2 96.68 ... 0.04891 0.04891 chi2_90 (freq) float32 2kB 153.0 149.0 138.3 ... 0.06995 0.06995 chi2_95 (freq) float32 2kB 199.1 194.0 180.0 ... 0.09105 0.09104 chi2_99 (freq) float32 2kB 306.0 298.1 276.5 ... 0.1399 0.1399 0.1399 Attributes: Description: Estimating red-noise spectra ... Input: OFAC = 1.0, HIFAC = 1.0, n50 ... Initial values: idum = -2.9221517e-36, Data v... Results: Avg. autocorr. coeff., rho = ... Equality of theoretical and data spectrum: 90-% acceptance region: rcrit... Elapsed time: 0 [s] About: Michael Schulz, Manfred Mudel... Reference: Schulz, M. and Mudelsee, M. (... Python platform: Shen yulu => https://github.c...
xarray.Dataset
- freq: 451
- freq(freq)float320.0 0.001111 ... 0.4989 0.5
array([0. , 0.001111, 0.002222, ..., 0.497778, 0.498889, 0.5 ], shape=(451,), dtype=float32)
- period(freq)float32inf 900.0 450.0 ... 2.009 2.004 2.0
array([ inf, 900. , 450. , 300. , 225. , 180. , 150. , 128.57143 , 112.5 , 100. , 90. , 81.81818 , 75. , 69.23077 , 64.28571 , 59.999996 , 56.25 , 52.941177 , 50. , 47.36842 , 45. , 42.857143 , 40.90909 , 39.130432 , 37.5 , 36. , 34.615383 , 33.333332 , 32.142857 , 31.034483 , 29.999998 , 29.032259 , 28.125 , 27.272726 , 26.470589 , 25.714285 , 25. , 24.324324 , 23.68421 , 23.076923 , 22.5 , 21.951218 , 21.428572 , 20.930231 , 20.454544 , 20. , 19.565216 , 19.148935 , 18.75 , 18.367346 , 18. , 17.647058 , 17.307692 , 16.981133 , 16.666666 , 16.363636 , 16.071428 , 15.789474 , 15.5172415, 15.254237 , 14.999999 , 14.754097 , 14.5161295, 14.285714 , 14.0625 , 13.846153 , 13.636363 , 13.432837 , 13.235294 , 13.043478 , 12.857142 , 12.676056 , 12.5 , 12.328767 , 12.162162 , 12. , 11.842105 , 11.688311 , 11.538462 , 11.392405 , 11.25 , 11.111111 , 10.975609 , 10.843373 , 10.714286 , 10.588235 , 10.465116 , 10.344827 , 10.227272 , 10.11236 , 10. , 9.89011 , 9.782608 , 9.677419 , 9.574468 , 9.473684 , 9.375 , 9.27835 , 9.183673 , 9.090909 , ... 2.535211 , 2.52809 , 2.5210083, 2.5139663, 2.5069637, 2.5 , 2.4930747, 2.4861877, 2.479339 , 2.4725275, 2.4657533, 2.4590163, 2.452316 , 2.445652 , 2.4390244, 2.4324324, 2.425876 , 2.4193547, 2.4128687, 2.4064171, 2.3999999, 2.393617 , 2.3872678, 2.3809524, 2.3746703, 2.368421 , 2.3622046, 2.356021 , 2.3498695, 2.34375 , 2.3376622, 2.3316061, 2.3255813, 2.3195875, 2.3136246, 2.3076923, 2.3017902, 2.2959182, 2.2900763, 2.2842638, 2.278481 , 2.2727273, 2.2670026, 2.2613065, 2.255639 , 2.25 , 2.244389 , 2.2388058, 2.2332506, 2.2277226, 2.222222 , 2.2167487, 2.2113023, 2.2058823, 2.2004888, 2.195122 , 2.189781 , 2.184466 , 2.1791768, 2.173913 , 2.1686747, 2.1634614, 2.1582735, 2.15311 , 2.1479712, 2.142857 , 2.137767 , 2.1327014, 2.1276596, 2.1226416, 2.117647 , 2.112676 , 2.1077282, 2.1028037, 2.097902 , 2.0930233, 2.088167 , 2.0833333, 2.078522 , 2.0737326, 2.0689654, 2.0642202, 2.0594966, 2.0547945, 2.050114 , 2.0454545, 2.0408163, 2.036199 , 2.0316026, 2.027027 , 2.022472 , 2.0179372, 2.0134227, 2.0089285, 2.0044544, 2. ], dtype=float32)
- period_month(freq)float32inf 75.0 37.5 ... 0.167 0.1667
array([ inf, 75. , 37.5 , 25. , 18.75 , 15. , 12.5 , 10.714286 , 9.375 , 8.333333 , 7.5 , 6.8181815 , 6.25 , 5.7692304 , 5.357143 , 4.9999995 , 4.6875 , 4.4117646 , 4.1666665 , 3.9473684 , 3.75 , 3.5714285 , 3.4090908 , 3.2608693 , 3.125 , 3. , 2.8846152 , 2.7777777 , 2.6785715 , 2.586207 , 2.4999998 , 2.419355 , 2.34375 , 2.2727273 , 2.2058823 , 2.142857 , 2.0833333 , 2.027027 , 1.9736842 , 1.923077 , 1.875 , 1.8292681 , 1.7857143 , 1.7441859 , 1.7045454 , 1.6666666 , 1.6304346 , 1.5957446 , 1.5625 , 1.5306121 , 1.5 , 1.4705882 , 1.4423076 , 1.4150944 , 1.3888888 , 1.3636364 , 1.3392857 , 1.3157895 , 1.2931035 , 1.2711865 , 1.2499999 , 1.229508 , 1.2096775 , 1.1904762 , 1.171875 , 1.1538461 , 1.1363636 , 1.119403 , 1.1029412 , 1.0869565 , 1.0714285 , 1.056338 , 1.0416666 , 1.0273973 , 1.0135134 , 1. , 0.9868421 , 0.9740259 , 0.9615385 , 0.94936705, 0.9375 , 0.9259259 , 0.91463405, 0.90361446, 0.89285713, 0.8823529 , 0.87209296, 0.8620689 , 0.8522727 , 0.84269667, 0.8333333 , 0.82417583, 0.8152173 , 0.80645156, 0.7978723 , 0.7894737 , 0.78125 , 0.7731958 , 0.76530606, 0.75757575, ... 0.21126759, 0.21067417, 0.21008402, 0.2094972 , 0.20891364, 0.20833333, 0.20775622, 0.2071823 , 0.20661157, 0.20604396, 0.20547944, 0.20491803, 0.20435967, 0.20380433, 0.20325203, 0.2027027 , 0.20215632, 0.20161289, 0.2010724 , 0.20053476, 0.19999999, 0.19946808, 0.19893898, 0.1984127 , 0.1978892 , 0.19736843, 0.19685037, 0.19633508, 0.19582246, 0.1953125 , 0.19480519, 0.19430052, 0.19379844, 0.19329895, 0.19280206, 0.1923077 , 0.19181585, 0.19132651, 0.1908397 , 0.19035532, 0.18987341, 0.18939394, 0.18891688, 0.18844222, 0.18796992, 0.1875 , 0.18703242, 0.18656714, 0.18610422, 0.18564355, 0.18518518, 0.18472905, 0.1842752 , 0.18382353, 0.18337406, 0.18292683, 0.18248175, 0.18203883, 0.18159807, 0.18115942, 0.18072289, 0.18028845, 0.17985612, 0.17942584, 0.17899759, 0.17857142, 0.17814726, 0.17772512, 0.17730497, 0.1768868 , 0.17647058, 0.17605633, 0.17564403, 0.17523365, 0.17482518, 0.17441861, 0.17401391, 0.1736111 , 0.17321016, 0.17281105, 0.17241378, 0.17201835, 0.17162472, 0.17123288, 0.17084283, 0.17045455, 0.17006803, 0.16968326, 0.16930021, 0.16891891, 0.16853933, 0.16816144, 0.16778523, 0.16741072, 0.16703786, 0.16666667], dtype=float32)
- gxx(freq)float321.603e-13 2.266 ... 0.008833
- Description :
- spectrum of input data
array([1.60316205e-13, 2.26551175e+00, 5.42321348e+00, 7.42832088e+00, 3.01133108e+00, 6.93038583e-01, 2.82339020e+01, 2.12355423e+01, 1.18938732e+01, 1.06654668e+00, 6.06137657e+00, 2.28831792e+00, 1.61298962e+01, 1.75784931e+01, 1.08234329e+01, 3.03243198e+01, 4.54110489e+01, 1.29023819e+01, 3.52281494e+01, 7.40147769e-01, 1.98424835e+01, 6.08881149e+01, 4.20661974e+00, 3.08798504e+00, 1.75222225e+01, 3.88681197e+00, 4.61709518e+01, 3.56018114e+00, 6.11290455e-01, 1.46126347e+01, 1.21116304e+01, 3.63837700e+01, 5.83606434e+00, 1.00964725e+00, 2.81207681e-01, 2.85525208e+01, 3.23619628e+00, 1.40037215e+00, 1.46946594e-01, 3.23534036e+00, 1.14253788e+01, 5.06294668e-01, 6.39495754e+00, 6.25039458e-01, 1.06484852e+01, 1.08395648e+00, 3.10801482e+00, 1.59288794e-01, 2.15409660e+00, 1.22570190e+01, 1.08592081e+01, 1.23673229e+01, 9.74308729e-01, 1.40486431e+00, 5.61834097e+00, 7.43666351e-01, 4.63068664e-01, 5.74096203e+00, 2.04190701e-01, 7.09333467e+00, 3.21358562e+00, 2.07995987e+00, 2.10914922e+00, 2.31298184e+00, 8.00730884e-02, 3.18285614e-01, 2.04544932e-01, 2.00223637e+00, 6.06358200e-02, 2.87699962e+00, 3.53316255e-02, 1.01997983e+00, 2.14095235e-01, 1.38981962e+00, 1.14668958e-01, 7.73526013e-01, 2.25469899e+00, 3.62235814e-01, 3.55819799e-03, 1.62805271e+00, ... 2.51763947e-02, 1.00441137e-02, 1.10441283e-03, 2.21373327e-03, 1.44165233e-02, 8.87131598e-03, 7.29839224e-03, 1.45069854e-02, 2.91344302e-04, 3.51019762e-03, 9.29261805e-05, 7.24822050e-03, 1.08684087e-02, 3.00684408e-03, 1.38091631e-02, 8.16676952e-03, 7.02145044e-03, 3.47863487e-03, 8.05350859e-03, 1.58347152e-02, 1.54852187e-02, 6.77592529e-04, 6.10266440e-03, 2.84996582e-03, 2.12125201e-02, 9.91427060e-03, 2.09325962e-02, 6.10002782e-03, 4.44939919e-02, 2.13280655e-02, 3.59338801e-03, 3.57864774e-03, 1.40766501e-02, 1.85679775e-02, 9.06299148e-03, 2.96612550e-03, 6.50333753e-03, 2.45106360e-03, 3.98470555e-03, 6.89184875e-04, 6.93784619e-04, 4.67400940e-04, 5.35062328e-03, 8.67869053e-03, 2.26087272e-02, 1.41341449e-03, 9.27686319e-03, 5.41148009e-03, 7.11101515e-04, 2.58170292e-02, 1.95200499e-02, 1.45601658e-02, 5.45096071e-03, 1.14019820e-02, 5.68486145e-03, 8.60104151e-03, 1.34293893e-02, 9.76453908e-03, 3.36495345e-03, 6.44261716e-04, 1.95370410e-02, 2.67344387e-03, 5.78412507e-03, 3.17232916e-03, 5.85582331e-02, 1.46740489e-02, 5.19550033e-03, 6.68154098e-03, 6.68761134e-03, 1.75048634e-02, 8.38467572e-03, 3.90124656e-02, 3.50213633e-03, 9.27943364e-03, 1.76406428e-02, 1.87265240e-02, 7.81876966e-03, 1.00313639e-02, 8.83288495e-03], dtype=float32)
- gxx_corr(freq)float324.176 3.245 ... 0.00842 0.007062
- Description :
- bias-corrected spectrum of input data
array([4.17632389e+00, 3.24510694e+00, 5.20200205e+00, 7.14972639e+00, 2.77049398e+00, 6.10014796e-01, 2.62322292e+01, 1.78901005e+01, 1.00870228e+01, 8.71064961e-01, 5.05846786e+00, 1.86555350e+00, 1.38912735e+01, 1.43095217e+01, 9.06318283e+00, 2.43293514e+01, 3.63216057e+01, 1.00131302e+01, 2.83889122e+01, 6.20126724e-01, 1.58653755e+01, 4.89103775e+01, 3.41352630e+00, 2.39977336e+00, 1.37498512e+01, 3.32375979e+00, 3.71273193e+01, 2.90647721e+00, 4.80742544e-01, 1.13361874e+01, 9.38038540e+00, 2.90714817e+01, 4.74376297e+00, 8.20160925e-01, 2.33650565e-01, 2.28395996e+01, 2.67142439e+00, 1.08217537e+00, 1.20632768e-01, 2.52920890e+00, 1.01443224e+01, 3.99443775e-01, 5.23169041e+00, 4.99116778e-01, 8.13497448e+00, 8.31849873e-01, 2.46893501e+00, 1.33835033e-01, 1.72858584e+00, 9.44733238e+00, 8.70027065e+00, 1.06336069e+01, 7.97397494e-01, 1.12997925e+00, 4.44095755e+00, 6.02594852e-01, 3.65704745e-01, 4.53237247e+00, 1.61730036e-01, 5.51169825e+00, 2.56679964e+00, 1.62108040e+00, 1.56782579e+00, 1.96369600e+00, 6.28718585e-02, 2.58172542e-01, 1.63326949e-01, 1.61752641e+00, 4.88981493e-02, 2.36317348e+00, 2.79120188e-02, 8.53541076e-01, 1.60275057e-01, 1.05810523e+00, 9.01982635e-02, 6.21759236e-01, 1.93588388e+00, 2.93866515e-01, 2.90891877e-03, 1.30646932e+00, ... 1.99714918e-02, 8.31583794e-03, 8.92210868e-04, 1.72990293e-03, 1.18730888e-02, 7.27409590e-03, 5.63104684e-03, 1.13333017e-02, 2.29926954e-04, 2.78377463e-03, 7.69944309e-05, 5.51623851e-03, 8.20089318e-03, 2.36387644e-03, 1.13241877e-02, 6.28952449e-03, 5.37202368e-03, 2.72377511e-03, 6.52828068e-03, 1.26013132e-02, 1.24996221e-02, 5.26369899e-04, 4.85904468e-03, 2.14236882e-03, 1.60741974e-02, 8.05601478e-03, 1.56529378e-02, 4.81670257e-03, 3.57378908e-02, 1.62757803e-02, 2.78253970e-03, 2.78204284e-03, 1.13680456e-02, 1.43720908e-02, 7.04678288e-03, 2.41956697e-03, 5.09034423e-03, 1.89686043e-03, 3.12023540e-03, 5.32147882e-04, 5.44103968e-04, 3.67142231e-04, 4.21444513e-03, 6.60895323e-03, 1.81989074e-02, 1.12454814e-03, 7.07757194e-03, 4.25253296e-03, 5.53195074e-04, 1.92246158e-02, 1.58733185e-02, 1.15699712e-02, 4.37291805e-03, 9.19797644e-03, 4.60562110e-03, 6.89818058e-03, 1.10857235e-02, 8.15438852e-03, 2.62143463e-03, 5.28839708e-04, 1.67828519e-02, 2.15478637e-03, 4.52012895e-03, 2.52539036e-03, 4.77485918e-02, 1.15080979e-02, 4.12895530e-03, 5.44822449e-03, 5.16246958e-03, 1.42008541e-02, 6.70567947e-03, 3.09495497e-02, 2.58457777e-03, 7.17677688e-03, 1.38893444e-02, 1.60318092e-02, 6.14564773e-03, 8.41981173e-03, 7.06179300e-03], dtype=float32)
- gred_th(freq)float3266.46 64.73 ... 0.03038 0.03038
- Description :
- theoretical AR(1) spectrum
array([6.64592743e+01, 6.47336349e+01, 6.00555267e+01, 5.36055946e+01, 4.65972252e+01, 3.98923874e+01, 3.39263916e+01, 2.88318272e+01, 2.45733871e+01, 2.10503502e+01, 1.81438751e+01, 1.57415657e+01, 1.37488737e+01, 1.20856714e+01, 1.06895733e+01, 9.50988770e+00, 8.50662518e+00, 7.64799452e+00, 6.90855074e+00, 6.26809406e+00, 5.71029997e+00, 5.22192717e+00, 4.79222679e+00, 4.41237450e+00, 4.07513952e+00, 3.77451992e+00, 3.50544715e+00, 3.26376963e+00, 3.04595852e+00, 2.84898257e+00, 2.67034173e+00, 2.50783587e+00, 2.35959673e+00, 2.22404122e+00, 2.09976602e+00, 1.98556590e+00, 1.88039315e+00, 1.78332841e+00, 1.69356811e+00, 1.61039925e+00, 1.53320312e+00, 1.46141756e+00, 1.39455748e+00, 1.33218598e+00, 1.27390802e+00, 1.21937919e+00, 1.16828537e+00, 1.12034500e+00, 1.07530642e+00, 1.03294063e+00, 9.93041158e-01, 9.55421567e-01, 9.19911504e-01, 8.86357486e-01, 8.54619205e-01, 8.24568629e-01, 7.96086371e-01, 7.69069016e-01, 7.43416071e-01, 7.19037652e-01, 6.95851862e-01, 6.73782349e-01, 6.52758300e-01, 6.32715225e-01, 6.13592565e-01, 5.95335782e-01, 5.77893734e-01, 5.61217606e-01, 5.45264840e-01, 5.29993236e-01, 5.15365005e-01, 5.01344323e-01, 4.87898707e-01, 4.74996835e-01, 4.62610304e-01, 4.50711071e-01, 4.39274967e-01, 4.28277791e-01, 4.17697936e-01, 4.07514095e-01, ... 3.27516161e-02, 3.26883011e-02, 3.26259620e-02, 3.25645879e-02, 3.25041860e-02, 3.24447379e-02, 3.23862359e-02, 3.23286913e-02, 3.22720744e-02, 3.22163925e-02, 3.21616381e-02, 3.21078040e-02, 3.20548825e-02, 3.20028737e-02, 3.19517702e-02, 3.19015644e-02, 3.18522491e-02, 3.18038277e-02, 3.17562856e-02, 3.17096226e-02, 3.16638313e-02, 3.16189118e-02, 3.15748528e-02, 3.15316543e-02, 3.14893126e-02, 3.14478241e-02, 3.14071774e-02, 3.13673727e-02, 3.13284136e-02, 3.12902816e-02, 3.12529802e-02, 3.12165059e-02, 3.11808567e-02, 3.11460234e-02, 3.11120078e-02, 3.10788024e-02, 3.10464092e-02, 3.10148224e-02, 3.09840366e-02, 3.09540480e-02, 3.09248567e-02, 3.08964625e-02, 3.08688525e-02, 3.08420341e-02, 3.08160018e-02, 3.07907481e-02, 3.07662785e-02, 3.07425875e-02, 3.07196714e-02, 3.06975264e-02, 3.06761507e-02, 3.06555498e-02, 3.06357089e-02, 3.06166336e-02, 3.05983257e-02, 3.05807777e-02, 3.05639859e-02, 3.05479541e-02, 3.05326767e-02, 3.05181537e-02, 3.05043887e-02, 3.04913744e-02, 3.04791071e-02, 3.04675866e-02, 3.04568224e-02, 3.04468013e-02, 3.04375254e-02, 3.04289963e-02, 3.04212123e-02, 3.04141697e-02, 3.04078721e-02, 3.04023139e-02, 3.03975027e-02, 3.03934291e-02, 3.03900968e-02, 3.03875059e-02, 3.03856581e-02, 3.03845461e-02, 3.03841773e-02], dtype=float32)
- gred(freq)float322.551e-12 45.19 ... 0.0362 0.038
- Description :
- average spectrum of Nsim AR(1) time series (uncorrected)
array([2.55116653e-12, 4.51926003e+01, 6.26093521e+01, 5.56943741e+01, 5.06478920e+01, 4.53217926e+01, 3.65151749e+01, 3.42233696e+01, 2.89751263e+01, 2.57744045e+01, 2.17411385e+01, 1.93088570e+01, 1.59645491e+01, 1.48466091e+01, 1.27657003e+01, 1.18532085e+01, 1.06353989e+01, 9.85479546e+00, 8.57290554e+00, 7.48123837e+00, 7.14174986e+00, 6.50073338e+00, 5.90564537e+00, 5.67776346e+00, 5.19318390e+00, 4.41393185e+00, 4.35931921e+00, 3.99783301e+00, 3.87310290e+00, 3.67241120e+00, 3.44785333e+00, 3.13862681e+00, 2.90291858e+00, 2.73787403e+00, 2.52715111e+00, 2.48221993e+00, 2.27793145e+00, 2.30768824e+00, 2.06298900e+00, 2.06000781e+00, 1.72682071e+00, 1.85234559e+00, 1.70463753e+00, 1.66828442e+00, 1.66751480e+00, 1.58893335e+00, 1.47069418e+00, 1.33342063e+00, 1.34000516e+00, 1.34014261e+00, 1.23946035e+00, 1.11119461e+00, 1.12400377e+00, 1.10197771e+00, 1.08119524e+00, 1.01760566e+00, 1.00803351e+00, 9.74146783e-01, 9.38592792e-01, 9.25372601e-01, 8.71193647e-01, 8.64509940e-01, 8.78136277e-01, 7.45257258e-01, 7.81466484e-01, 7.33954251e-01, 7.23733842e-01, 6.94696784e-01, 6.76151991e-01, 6.45229995e-01, 6.52360022e-01, 5.99105418e-01, 6.51734531e-01, 6.23907626e-01, 5.88116050e-01, 5.60726285e-01, 5.11617839e-01, 5.27918458e-01, 5.10929346e-01, 5.07822454e-01, ... 4.12872359e-02, 3.94818895e-02, 4.03856710e-02, 4.16724607e-02, 3.94671783e-02, 3.95688377e-02, 4.19757590e-02, 4.13817465e-02, 4.08924893e-02, 4.06232253e-02, 3.88165452e-02, 4.21889722e-02, 4.24814187e-02, 4.07075621e-02, 3.89632508e-02, 4.14232761e-02, 4.16321680e-02, 4.06178571e-02, 3.91756296e-02, 3.98460701e-02, 3.92268971e-02, 4.07028198e-02, 3.96560952e-02, 4.19461578e-02, 4.15552743e-02, 3.87017950e-02, 4.20006625e-02, 3.97246554e-02, 3.90041545e-02, 4.10033278e-02, 4.03602794e-02, 4.01549786e-02, 3.86101604e-02, 4.02390063e-02, 4.00136970e-02, 3.80992256e-02, 3.96643616e-02, 4.00763825e-02, 3.95682529e-02, 4.00885977e-02, 3.94321494e-02, 3.93336266e-02, 3.91908251e-02, 4.05008867e-02, 3.82830985e-02, 3.87000702e-02, 4.03266214e-02, 3.91208977e-02, 3.94884311e-02, 4.12241742e-02, 3.77236791e-02, 3.85783091e-02, 3.81882414e-02, 3.79529446e-02, 3.77684683e-02, 3.81298438e-02, 3.70256044e-02, 3.65798958e-02, 3.91926765e-02, 3.71789001e-02, 3.55103798e-02, 3.78306545e-02, 3.90021987e-02, 3.82725857e-02, 3.73518392e-02, 3.88229080e-02, 3.82998027e-02, 3.73172164e-02, 3.94085087e-02, 3.74904126e-02, 3.80215235e-02, 3.83226648e-02, 4.11890075e-02, 3.92981134e-02, 3.85979936e-02, 3.54952104e-02, 3.86580043e-02, 3.62001508e-02, 3.80045027e-02], dtype=float32)
- corrFac(freq)float323.839e-14 0.6981 ... 1.191 1.251
- Description :
- Gxx / Gxx_corr
array([3.8386917e-14, 6.9813162e-01, 1.0425243e+00, 1.0389657e+00, 1.0869293e+00, 1.1361012e+00, 1.0763059e+00, 1.1869997e+00, 1.1791263e+00, 1.2244169e+00, 1.1982633e+00, 1.2266160e+00, 1.1611532e+00, 1.2284472e+00, 1.1942198e+00, 1.2464089e+00, 1.2502489e+00, 1.2885463e+00, 1.2409122e+00, 1.1935427e+00, 1.2506785e+00, 1.2448915e+00, 1.2323384e+00, 1.2867819e+00, 1.2743572e+00, 1.1694021e+00, 1.2435843e+00, 1.2249128e+00, 1.2715547e+00, 1.2890255e+00, 1.2911656e+00, 1.2515279e+00, 1.2302605e+00, 1.2310356e+00, 1.2035395e+00, 1.2501322e+00, 1.2114123e+00, 1.2940344e+00, 1.2181317e+00, 1.2791907e+00, 1.1262830e+00, 1.2674992e+00, 1.2223501e+00, 1.2522910e+00, 1.3089758e+00, 1.3030674e+00, 1.2588484e+00, 1.1901876e+00, 1.2461612e+00, 1.2974052e+00, 1.2481461e+00, 1.1630411e+00, 1.2218608e+00, 1.2432655e+00, 1.2651192e+00, 1.2341067e+00, 1.2662364e+00, 1.2666572e+00, 1.2625403e+00, 1.2869599e+00, 1.2519815e+00, 1.2830701e+00, 1.3452702e+00, 1.1778716e+00, 1.2735919e+00, 1.2328408e+00, 1.2523649e+00, 1.2378385e+00, 1.2400433e+00, 1.2174306e+00, 1.2658212e+00, 1.1949979e+00, 1.3357989e+00, 1.3134985e+00, 1.2712990e+00, 1.2440925e+00, 1.1646870e+00, 1.2326542e+00, 1.2232029e+00, 1.2461469e+00, ... 1.2606167e+00, 1.2078294e+00, 1.2378384e+00, 1.2796865e+00, 1.2142184e+00, 1.2195765e+00, 1.2960987e+00, 1.2800317e+00, 1.2671168e+00, 1.2609489e+00, 1.2069207e+00, 1.3139788e+00, 1.3252714e+00, 1.2719971e+00, 1.2194395e+00, 1.2984717e+00, 1.3070401e+00, 1.2771373e+00, 1.2336339e+00, 1.2565924e+00, 1.2388550e+00, 1.2872934e+00, 1.2559391e+00, 1.3302872e+00, 1.3196628e+00, 1.2306669e+00, 1.3372951e+00, 1.2664323e+00, 1.2450089e+00, 1.3104173e+00, 1.2914058e+00, 1.2863380e+00, 1.2382648e+00, 1.2919468e+00, 1.2861174e+00, 1.2258910e+00, 1.2775830e+00, 1.2921686e+00, 1.2770529e+00, 1.2951003e+00, 1.2750957e+00, 1.2730787e+00, 1.2695913e+00, 1.3131716e+00, 1.2423123e+00, 1.2568733e+00, 1.3107409e+00, 1.2725310e+00, 1.2854444e+00, 1.3429152e+00, 1.2297397e+00, 1.2584444e+00, 1.2465271e+00, 1.2396185e+00, 1.2343311e+00, 1.2468566e+00, 1.2114129e+00, 1.1974581e+00, 1.2836305e+00, 1.2182553e+00, 1.1641073e+00, 1.2407001e+00, 1.2796372e+00, 1.2561738e+00, 1.2263865e+00, 1.2751063e+00, 1.2583086e+00, 1.2263703e+00, 1.2954286e+00, 1.2326627e+00, 1.2503842e+00, 1.2605181e+00, 1.3550129e+00, 1.2929806e+00, 1.2700846e+00, 1.1680856e+00, 1.2722450e+00, 1.1914001e+00, 1.2507992e+00], dtype=float32)
- chi2_80(freq)float32107.0 104.2 ... 0.04891 0.04891
- Description :
- 80-% false-alarm level (Chi^2)
array([1.06986290e+02, 1.04208351e+02, 9.66775208e+01, 8.62944031e+01, 7.50123215e+01, 6.42188568e+01, 5.46147804e+01, 4.64135399e+01, 3.95582924e+01, 3.38869019e+01, 2.92080517e+01, 2.53408070e+01, 2.21329670e+01, 1.94555416e+01, 1.72080994e+01, 1.53090382e+01, 1.36939840e+01, 1.23117590e+01, 1.11214008e+01, 1.00903921e+01, 9.19245338e+00, 8.40627003e+00, 7.71453762e+00, 7.10305023e+00, 6.56016874e+00, 6.07623053e+00, 5.64307690e+00, 5.25402355e+00, 4.90339088e+00, 4.58629847e+00, 4.29872227e+00, 4.03711987e+00, 3.79848409e+00, 3.58026648e+00, 3.38020802e+00, 3.19636846e+00, 3.02706122e+00, 2.87080598e+00, 2.72630978e+00, 2.59242439e+00, 2.46815395e+00, 2.35259318e+00, 2.24496174e+00, 2.14455605e+00, 2.05074000e+00, 1.96295941e+00, 1.88070846e+00, 1.80353391e+00, 1.73103070e+00, 1.66283011e+00, 1.59859991e+00, 1.53803980e+00, 1.48087561e+00, 1.42686021e+00, 1.37576795e+00, 1.32739246e+00, 1.28154159e+00, 1.23804903e+00, 1.19675291e+00, 1.15750837e+00, 1.12018394e+00, 1.08465636e+00, 1.05081177e+00, 1.01854634e+00, 9.87762690e-01, 9.58372891e-01, 9.30294633e-01, 9.03449357e-01, 8.77768576e-01, 8.53184283e-01, 8.29635739e-01, 8.07065189e-01, 7.85420418e-01, 7.64651000e-01, 7.44711101e-01, 7.25555718e-01, 7.07145810e-01, 6.89442575e-01, 6.72411084e-01, 6.56017125e-01, ... 5.27236238e-02, 5.26216999e-02, 5.25213480e-02, 5.24225459e-02, 5.23253120e-02, 5.22296131e-02, 5.21354340e-02, 5.20428009e-02, 5.19516580e-02, 5.18620200e-02, 5.17738760e-02, 5.16872145e-02, 5.16020209e-02, 5.15182987e-02, 5.14360331e-02, 5.13552092e-02, 5.12758233e-02, 5.11978716e-02, 5.11213392e-02, 5.10462224e-02, 5.09725064e-02, 5.09001948e-02, 5.08292690e-02, 5.07597290e-02, 5.06915674e-02, 5.06247766e-02, 5.05593456e-02, 5.04952669e-02, 5.04325517e-02, 5.03711663e-02, 5.03111184e-02, 5.02524003e-02, 5.01950122e-02, 5.01389392e-02, 5.00841811e-02, 5.00307269e-02, 4.99785803e-02, 4.99277301e-02, 4.98781726e-02, 4.98298965e-02, 4.97829020e-02, 4.97371964e-02, 4.96927463e-02, 4.96495776e-02, 4.96076681e-02, 4.95670140e-02, 4.95276228e-02, 4.94894870e-02, 4.94525954e-02, 4.94169481e-02, 4.93825376e-02, 4.93493713e-02, 4.93174344e-02, 4.92867269e-02, 4.92572524e-02, 4.92290035e-02, 4.92019728e-02, 4.91761640e-02, 4.91515733e-02, 4.91281934e-02, 4.91060317e-02, 4.90850843e-02, 4.90653366e-02, 4.90467884e-02, 4.90294620e-02, 4.90133278e-02, 4.89983968e-02, 4.89846654e-02, 4.89721373e-02, 4.89607975e-02, 4.89506610e-02, 4.89417128e-02, 4.89339679e-02, 4.89274114e-02, 4.89220470e-02, 4.89178747e-02, 4.89149019e-02, 4.89131100e-02, 4.89125177e-02], dtype=float32)
- chi2_90(freq)float32153.0 149.0 ... 0.06995 0.06995
- Description :
- 90-% false-alarm level (Chi^2)
array([1.53000534e+02, 1.49027817e+02, 1.38258026e+02, 1.23409180e+02, 1.07274727e+02, 9.18390503e+01, 7.81043167e+01, 6.63757629e+01, 5.65721092e+01, 4.84614792e+01, 4.17702827e+01, 3.62397575e+01, 3.16522427e+01, 2.78232670e+01, 2.46092129e+01, 2.18933754e+01, 1.95836964e+01, 1.76069813e+01, 1.59046564e+01, 1.44302168e+01, 1.31460800e+01, 1.20217628e+01, 1.10325193e+01, 1.01580353e+01, 9.38166332e+00, 8.68958569e+00, 8.07013416e+00, 7.51375198e+00, 7.01231384e+00, 6.55884171e+00, 6.14758015e+00, 5.77346420e+00, 5.43219233e+00, 5.12012053e+00, 4.83401775e+00, 4.57110977e+00, 4.32898426e+00, 4.10552502e+00, 3.89888144e+00, 3.70741248e+00, 3.52969384e+00, 3.36443138e+00, 3.21050811e+00, 3.06691837e+00, 2.93275261e+00, 2.80721784e+00, 2.68959141e+00, 2.57922435e+00, 2.47553802e+00, 2.37800479e+00, 2.28614926e+00, 2.19954276e+00, 2.11779237e+00, 2.04054546e+00, 1.96747851e+00, 1.89829695e+00, 1.83272600e+00, 1.77052748e+00, 1.71147001e+00, 1.65534675e+00, 1.60196912e+00, 1.55116141e+00, 1.50276041e+00, 1.45661783e+00, 1.41259432e+00, 1.37056410e+00, 1.33040953e+00, 1.29201818e+00, 1.25529230e+00, 1.22013438e+00, 1.18645775e+00, 1.15417981e+00, 1.12322569e+00, 1.09352338e+00, 1.06500745e+00, 1.03761339e+00, 1.01128554e+00, 9.85968173e-01, 9.61611569e-01, 9.38166618e-01, ... 7.53997788e-02, 7.52540231e-02, 7.51105025e-02, 7.49692097e-02, 7.48301521e-02, 7.46932924e-02, 7.45586157e-02, 7.44261369e-02, 7.42957965e-02, 7.41676092e-02, 7.40415528e-02, 7.39176199e-02, 7.37957805e-02, 7.36760497e-02, 7.35583976e-02, 7.34428167e-02, 7.33292848e-02, 7.32178092e-02, 7.31083602e-02, 7.30009377e-02, 7.28955194e-02, 7.27921054e-02, 7.26906732e-02, 7.25912228e-02, 7.24937469e-02, 7.23982304e-02, 7.23046586e-02, 7.22130165e-02, 7.21233264e-02, 7.20355436e-02, 7.19496682e-02, 7.18657002e-02, 7.17836246e-02, 7.17034340e-02, 7.16251284e-02, 7.15486780e-02, 7.14741051e-02, 7.14013875e-02, 7.13305101e-02, 7.12614730e-02, 7.11942688e-02, 7.11289048e-02, 7.10653365e-02, 7.10036010e-02, 7.09436685e-02, 7.08855316e-02, 7.08291978e-02, 7.07746595e-02, 7.07219020e-02, 7.06709176e-02, 7.06217065e-02, 7.05742836e-02, 7.05286041e-02, 7.04846904e-02, 7.04425424e-02, 7.04021454e-02, 7.03634843e-02, 7.03265741e-02, 7.02914074e-02, 7.02579692e-02, 7.02262819e-02, 7.01963231e-02, 7.01680779e-02, 7.01415613e-02, 7.01167732e-02, 7.00937063e-02, 7.00723529e-02, 7.00527132e-02, 7.00347945e-02, 7.00185820e-02, 7.00040832e-02, 6.99912906e-02, 6.99802116e-02, 6.99708313e-02, 6.99631646e-02, 6.99571967e-02, 6.99529424e-02, 6.99503869e-02, 6.99495375e-02], dtype=float32)
- chi2_95(freq)float32199.1 194.0 ... 0.09105 0.09104
- Description :
- 95-% false-alarm level (Chi^2)
array([1.99141541e+02, 1.93970764e+02, 1.79953064e+02, 1.60626205e+02, 1.39626007e+02, 1.19535332e+02, 1.01658554e+02, 8.63929749e+01, 7.36327972e+01, 6.30762100e+01, 5.43671188e+01, 4.71687317e+01, 4.11977386e+01, 3.62140465e+01, 3.20307159e+01, 2.84958534e+01, 2.54896317e+01, 2.29167919e+01, 2.07010899e+01, 1.87819977e+01, 1.71105976e+01, 1.56472158e+01, 1.43596430e+01, 1.32214365e+01, 1.22109299e+01, 1.13101406e+01, 1.05038786e+01, 9.77970505e+00, 9.12704659e+00, 8.53681850e+00, 8.00153160e+00, 7.51459169e+00, 7.07040119e+00, 6.66421652e+00, 6.29183292e+00, 5.94963837e+00, 5.63449430e+00, 5.34364510e+00, 5.07468319e+00, 4.82547235e+00, 4.59415817e+00, 4.37905693e+00, 4.17871428e+00, 3.99182153e+00, 3.81719494e+00, 3.65380239e+00, 3.50070262e+00, 3.35705185e+00, 3.22209620e+00, 3.09514952e+00, 2.97559285e+00, 2.86286783e+00, 2.75646400e+00, 2.65592122e+00, 2.56081915e+00, 2.47077441e+00, 2.38542891e+00, 2.30447268e+00, 2.22760510e+00, 2.15455651e+00, 2.08508158e+00, 2.01895165e+00, 1.95595419e+00, 1.89589620e+00, 1.83859622e+00, 1.78389072e+00, 1.73162663e+00, 1.68165755e+00, 1.63385594e+00, 1.58809543e+00, 1.54426277e+00, 1.50225055e+00, 1.46196151e+00, 1.42330170e+00, 1.38618624e+00, 1.35053074e+00, 1.31626320e+00, 1.28331077e+00, 1.25160873e+00, 1.22109342e+00, ... 9.81384069e-02, 9.79486853e-02, 9.77618918e-02, 9.75779891e-02, 9.73969996e-02, 9.72188637e-02, 9.70435664e-02, 9.68711376e-02, 9.67014879e-02, 9.65346396e-02, 9.63705704e-02, 9.62092578e-02, 9.60506797e-02, 9.58948433e-02, 9.57417116e-02, 9.55912769e-02, 9.54435021e-02, 9.52984095e-02, 9.51559544e-02, 9.50161293e-02, 9.48789194e-02, 9.47443172e-02, 9.46123004e-02, 9.44828615e-02, 9.43559855e-02, 9.42316651e-02, 9.41098705e-02, 9.39905941e-02, 9.38738585e-02, 9.37595963e-02, 9.36478302e-02, 9.35385302e-02, 9.34317112e-02, 9.33273360e-02, 9.32254121e-02, 9.31259096e-02, 9.30288509e-02, 9.29341987e-02, 9.28419530e-02, 9.27520916e-02, 9.26646218e-02, 9.25795436e-02, 9.24968123e-02, 9.24164504e-02, 9.23384428e-02, 9.22627747e-02, 9.21894535e-02, 9.21184644e-02, 9.20497999e-02, 9.19834375e-02, 9.19193923e-02, 9.18576568e-02, 9.17982087e-02, 9.17410478e-02, 9.16861892e-02, 9.16336104e-02, 9.15832967e-02, 9.15352553e-02, 9.14894789e-02, 9.14459601e-02, 9.14047137e-02, 9.13657174e-02, 9.13289562e-02, 9.12944376e-02, 9.12621841e-02, 9.12321582e-02, 9.12043601e-02, 9.11788046e-02, 9.11554769e-02, 9.11343768e-02, 9.11155045e-02, 9.10988525e-02, 9.10844356e-02, 9.10722315e-02, 9.10622478e-02, 9.10544842e-02, 9.10489410e-02, 9.10456106e-02, 9.10445079e-02], dtype=float32)
- chi2_99(freq)float32306.0 298.1 276.5 ... 0.1399 0.1399
- Description :
- 99-% false-alarm level (Chi^2)
array([3.0600107e+02, 2.9805563e+02, 2.7651605e+02, 2.4681836e+02, 2.1454945e+02, 1.8367810e+02, 1.5620863e+02, 1.3275153e+02, 1.1314422e+02, 9.6922958e+01, 8.3540565e+01, 7.2479515e+01, 6.3304485e+01, 5.5646534e+01, 4.9218426e+01, 4.3786751e+01, 3.9167393e+01, 3.5213963e+01, 3.1809313e+01, 2.8860434e+01, 2.6292160e+01, 2.4043526e+01, 2.2065039e+01, 2.0316071e+01, 1.8763327e+01, 1.7379171e+01, 1.6140268e+01, 1.5027504e+01, 1.4024628e+01, 1.3117683e+01, 1.2295160e+01, 1.1546928e+01, 1.0864385e+01, 1.0240241e+01, 9.6680355e+00, 9.1422195e+00, 8.6579685e+00, 8.2110500e+00, 7.7977629e+00, 7.4148250e+00, 7.0593877e+00, 6.7288628e+00, 6.4210162e+00, 6.1338367e+00, 5.8655052e+00, 5.6144357e+00, 5.3791828e+00, 5.1584487e+00, 4.9510760e+00, 4.7560096e+00, 4.5722985e+00, 4.3990855e+00, 4.2355847e+00, 4.0810909e+00, 3.9349570e+00, 3.7965939e+00, 3.6654520e+00, 3.5410550e+00, 3.4229400e+00, 3.3106935e+00, 3.2039382e+00, 3.1023228e+00, 3.0055208e+00, 2.9132357e+00, 2.8251886e+00, 2.7411282e+00, 2.6608191e+00, 2.5840364e+00, 2.5105846e+00, 2.4402688e+00, 2.3729155e+00, 2.3083596e+00, 2.2464514e+00, 2.1870468e+00, 2.1300149e+00, 2.0752268e+00, 2.0225711e+00, 1.9719363e+00, 1.9232231e+00, 1.8763332e+00, ... 1.5079956e-01, 1.5050805e-01, 1.5022101e-01, 1.4993842e-01, 1.4966030e-01, 1.4938658e-01, 1.4911723e-01, 1.4885227e-01, 1.4859159e-01, 1.4833522e-01, 1.4808311e-01, 1.4783524e-01, 1.4759156e-01, 1.4735210e-01, 1.4711680e-01, 1.4688563e-01, 1.4665857e-01, 1.4643562e-01, 1.4621672e-01, 1.4600188e-01, 1.4579104e-01, 1.4558421e-01, 1.4538135e-01, 1.4518245e-01, 1.4498749e-01, 1.4479646e-01, 1.4460932e-01, 1.4442603e-01, 1.4424665e-01, 1.4407109e-01, 1.4389934e-01, 1.4373140e-01, 1.4356725e-01, 1.4340687e-01, 1.4325026e-01, 1.4309736e-01, 1.4294821e-01, 1.4280277e-01, 1.4266102e-01, 1.4252295e-01, 1.4238854e-01, 1.4225781e-01, 1.4213067e-01, 1.4200720e-01, 1.4188734e-01, 1.4177106e-01, 1.4165840e-01, 1.4154932e-01, 1.4144380e-01, 1.4134184e-01, 1.4124341e-01, 1.4114857e-01, 1.4105721e-01, 1.4096938e-01, 1.4088508e-01, 1.4080429e-01, 1.4072697e-01, 1.4065315e-01, 1.4058281e-01, 1.4051594e-01, 1.4045256e-01, 1.4039265e-01, 1.4033616e-01, 1.4028312e-01, 1.4023355e-01, 1.4018741e-01, 1.4014471e-01, 1.4010543e-01, 1.4006959e-01, 1.4003716e-01, 1.4000817e-01, 1.3998258e-01, 1.3996042e-01, 1.3994166e-01, 1.3992633e-01, 1.3991439e-01, 1.3990588e-01, 1.3990077e-01, 1.3989908e-01], dtype=float32)
- freqPandasIndex
PandasIndex(Index([ 0.0, 0.0011111111380159855, 0.002222222276031971, 0.0033333334140479565, 0.004444444552063942, 0.0055555556900799274, 0.006666666828095913, 0.007777777966111898, 0.008888889104127884, 0.009999999776482582, ... 0.49000000953674316, 0.4911111295223236, 0.49222221970558167, 0.4933333396911621, 0.49444445967674255, 0.495555579662323, 0.49666666984558105, 0.4977777898311615, 0.49888890981674194, 0.5], dtype='float32', name='freq', length=451))
- Description :
- Estimating red-noise spectra directly from unevenly spaced paleoclimatic time series.
- Input :
- OFAC = 1.0, HIFAC = 1.0, n50 = 1, Iwin = 0, Nsim = 1000
- Initial values :
- idum = -2.9221517e-36, Data variance (from data spectrum) = 0.74743754, Avg. dt = 1.0
- Results :
- Avg. autocorr. coeff., rho = 0.95813054, Avg. tau = 23.38019, Degrees of freedom = 2.0, 6-dB Bandwidth = 0.0013444445, Critical false-alarm level (Thomson, 1990) = 99.888885, ==> corresponding scaling factor for red noise = 6.801605
- Equality of theoretical and data spectrum :
- 90-% acceptance region: rcritlo = 2.91e-43, rcrithi = 3.4e-43; 95-% acceptance region: rcritlo = 2.87e-43, rcrithi = 3.45e-43; 98-% acceptance region: rcritlo = 2.82e-43, rcrithi = 3.52e-43; r_test = 161
- Elapsed time :
- 0 [s]
- About :
- Michael Schulz, Manfred Mudelsee => https://www.marum.de/Prof.-Dr.-michael-schulz/Michael-Schulz-Software.html
- Reference :
- Schulz, M. and Mudelsee, M. (2002) REDFIT: Estimating red-noise spectra directly from unevenly spaced paleoclimatic time series. Computers and Geosciences, 28, 421-426. https://doi.org/10.1016/S0098-3004(01)00044-9
- Python platform :
- Shen yulu => https://github.com/shenyulu/easyclimate-backend
Draw the red noise graph
fig, ax = plt.subplots()
result_redfit.gxx.plot(ax = ax, x = 'period_month', color = 'black')
result_redfit.chi2_95.plot(ax = ax, ls = '--', x = 'period_month', label = '95% CI', color = 'red')
result_redfit.chi2_90.plot(ax = ax, ls = '--', x = 'period_month', label = '90% CI', color = 'blue')
ax.legend()
ax.set_xscale('log', base = 2, subs = None)
ax.xaxis.set_major_formatter(ticker.ScalarFormatter())
ax.set_xlim(2, 16)
ax.set_xlabel('Period (years)')
ax.set_ylabel('Spectral Amplitude')
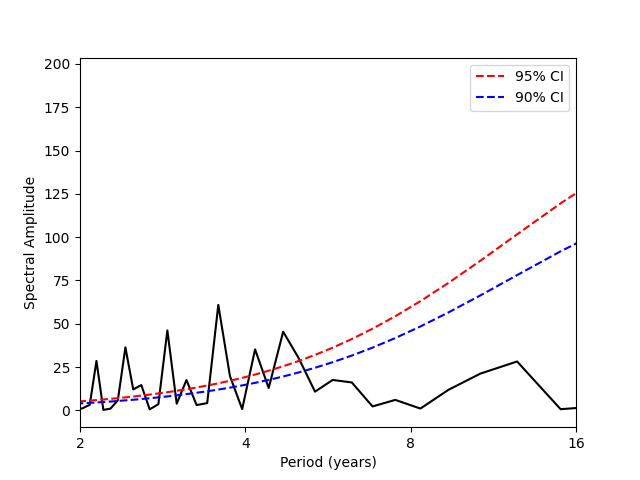
Text(38.347222222222214, 0.5, 'Spectral Amplitude')
Total running time of the script: (0 minutes 0.679 seconds)