Note
Go to the end to download the full example code.
Barnes Filter#
Barnes filter is a commonly used spatial filtering method that mainly uses two constants g and c to calculate Gaussian weights, and performs spatial interpolation for each grid point, thus becoming a low-pass filter that filters out high-frequency fluctuations. When using two different schemes of constant g and c schemes, both retain low-frequency fluctuations of different scales. The difference between the filtering results of the two methods can result in mesoscale fluctuations.
See also
Maddox, R. A. (1980). An Objective Technique for Separating Macroscale and Mesoscale Features in Meteorological Data. Monthly Weather Review, 108(8), 1108-1121. https://journals.ametsoc.org/view/journals/mwre/108/8/1520-0493_1980_108_1108_aotfsm_2_0_co_2.xml
Before proceeding with all the steps, first import some necessary libraries and packages
import matplotlib.pyplot as plt
import easyclimate as ecl
Open tuturial dataset
hgt_data = ecl.open_tutorial_dataset("hgt_2022_day5").hgt.sel(level = 500)
hgt_data
Filter dataset using easyclimate.filter.calc_barnes_lowpass
Caculating the first revision...
Caculating the second revision...
Draw results and differences.
fig, ax = plt.subplots(3,1, figsize = (5, 15))
axi = ax[0]
hgt_data.isel(time = 0).plot.contourf(
ax = axi, levels = 21,
cbar_kwargs={"location": "right", "aspect": 30},
)
axi.set_title("Raw data")
axi = ax[1]
hgt_data1.isel(time = 0).plot.contourf(
ax = axi, levels = 21,
cbar_kwargs={"location": "right", "aspect": 30},
)
axi.set_title("Filtered data")
axi = ax[2]
draw_dta = hgt_data.isel(time = 0) - hgt_data1.isel(time = 0)
draw_dta.plot.contourf(
ax = axi, levels = 21,
cbar_kwargs={"location": "right", "aspect": 30},
)
axi.set_title("Mesoscale fluctuations")
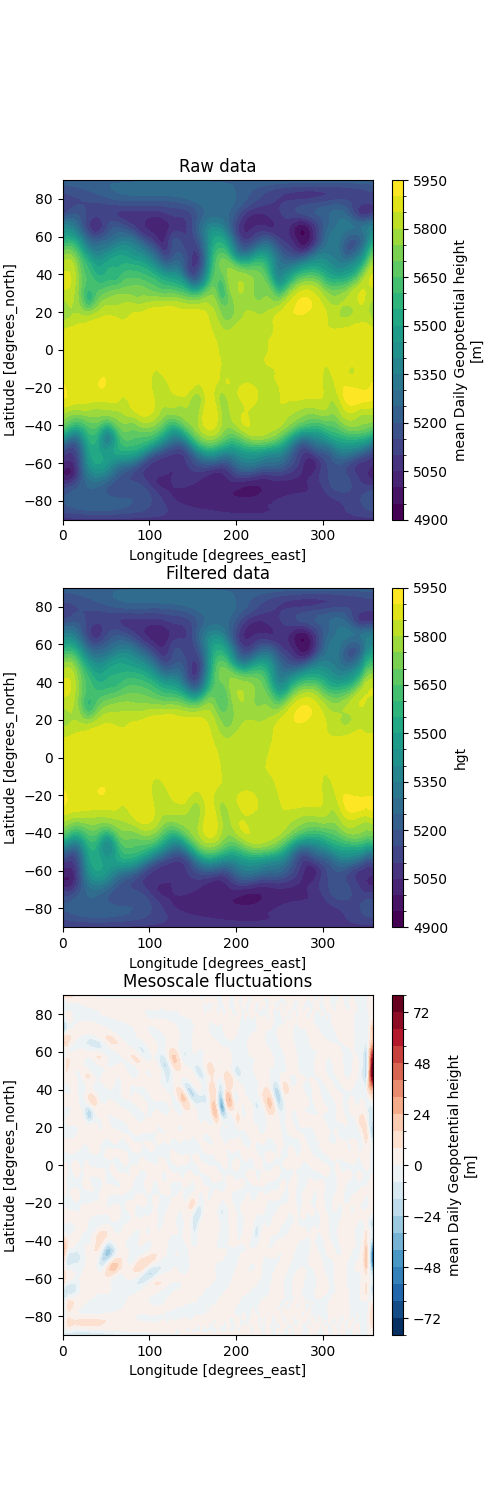
Text(0.5, 1.0, 'Mesoscale fluctuations')
Total running time of the script: (0 minutes 1.713 seconds)